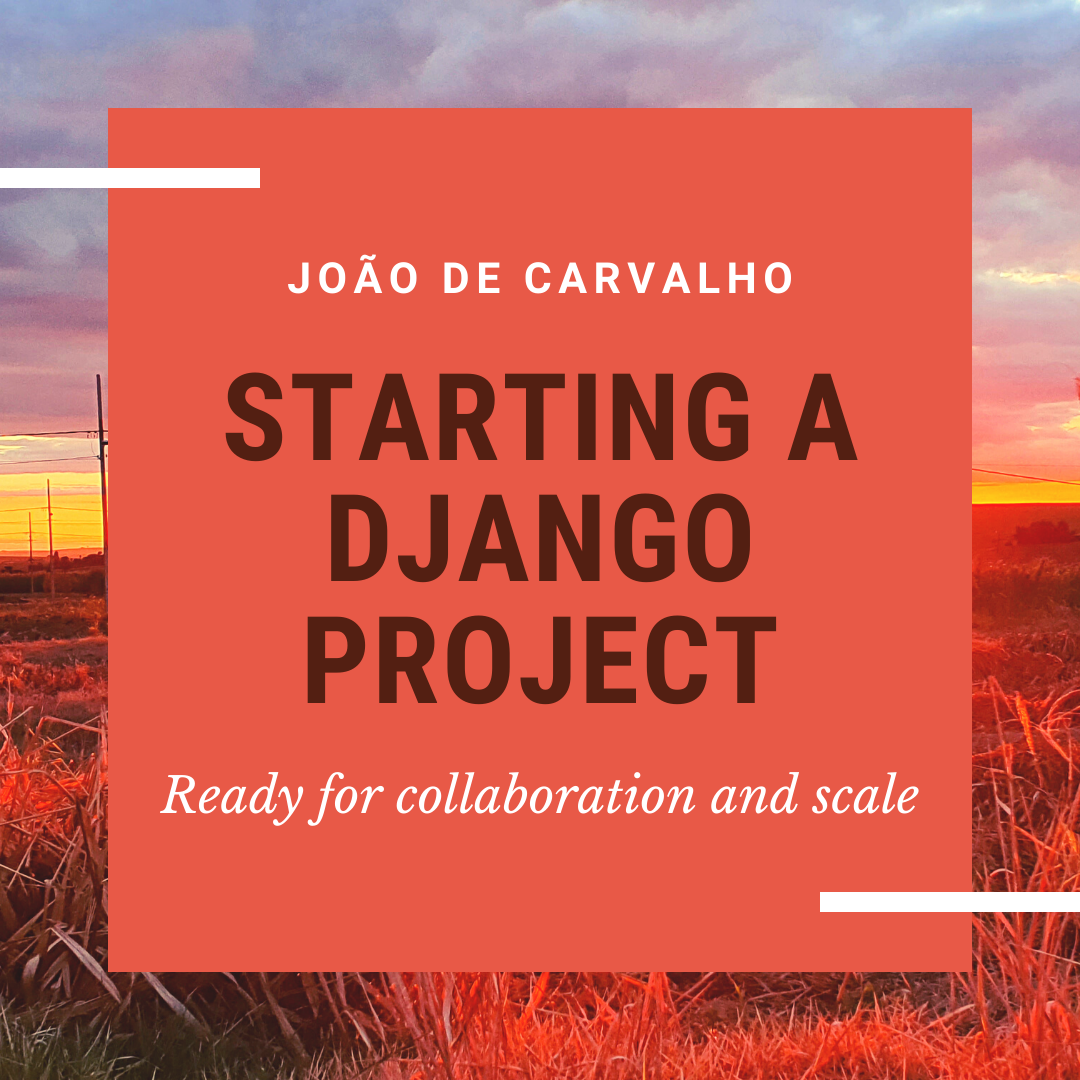
Starting a Django project ready for collaboration and scale
There are some things that I do when starting a new Django framework project. Share these things, maybe can help other developers to achieve success in their projects, so let's do it!
1 - Have a good project directory structure:
A good directory structure helps you to handle sources and dependencies, personally, I use the structure below:
All my source codes will live in the src subfolder, meanwhile, a subfolder for the virtual environment will live on its side. Doing this way keeps the environment easily findable and will avoid one more line at the .gitignore file.
2 - Always use a virtual environment:
It's usual for Python projects to use virtual environments, but it doesn't hurt to talk. A virtual environment is a sandbox that isolates your app dependencies from the outside world. For example, if ten different Python projects are running in the same container, each one needing different Python and library versions, without an isolated environment for each application, those interpreter and library versions will clash sometimes and crash apps.
To create and activate a new virtualenv environment just type:
Another good practice is creating a working alias for the project, doing this at the .bash_aliases file.
3 - Keep sensitive data out of the repository:
The Django settings.py file has sensitive data everywhere. It is a good idea to put those data as environment variables for two main reasons:
- The data usually changes according to running's places;
- Sensitive data must be hidden;
To do that, we must have two files: env.py and env.sample.py.
- The env.py file will be responsible for containing all variables with sensitive data and must stay out of the repository;
- The env.sample.py will contain all variables without their values, working as a sample for incoming developers and staying tracked at the repo;
First of all, create the env.sample.py file:
So, let's create the .gitignore file
The .gitgnore file must contain instructions that ignore the env.py file addition to the repository.
Now we must do two things:
- To copy env.sample.py as env.py and fill our variables with the sensitive data;
- To load those variables create a script in project_name/__init__.py
Finally, the only thing left is to replace the sensitive data with the variables:
4 - Choose Python and Django Framework version wisely:
On the Django site download page, the image below shows the project's version roadmap:
So, those are some thoughts of mine about this:
- Python 2. x is gone. The support for this Python version ended with the Django 1.11 LTS;
- Please keep ALL DEV TEAM with the same Python 3. x version;
- ALWAYS prefer the last long-term support version;
- ALWAYS keep one eye on your Django version's support ending date;
5 - Create a strong .gitignore policy:
This is a common .gitignore file for my Django projects.
Everything In the project that may cause mess or misunderstanding at deployment time must lie here:
Things such as:
- Environment variables file with sensitive data;
- Compiled C python files;
- All collected media and static files;
- IDE workspace settings files;
- Cache files and cache folders generated by running Python projects;
- Migration files;
Many people keep migration files in the repository, but not me. All generated source code must be kept out of the repository, which includes migration files.
The official docs to the Django migration files, however, recommend another point of view:
The migration files for each app live in a “migrations” directory inside of that app, and are designed to be committed to, and distributed as part of, its codebase. You should be making them once on your development machine and then running the same migrations on your colleagues’ machines, your staging machines, and eventually your production machines.
But, If you, for example, have a FileField on your Model class and the Django application has to migrate hundreds of databases for each customer, the migration file for this field will include the absolute path to the developer workspace. For that reason, even if the developer does not change the model file, the project still will generate a new migration file when another developer runs the make migrations command. This only can be fixed with a merge operation and running the migrate command again.
Obviously, this issue doesn't occur when all developers have their development workspaces in a development container or VM. But for me, production applications go better when migration files are created at deployment time.
These are my tips for starting a Django project with the aim of scalability for production-ready projects.